In the previous lesson, we learn about the different kind of IDEs that exist for android development. Now, we will be using Android Studio to create a project and review each file and folder created, and thinks to consider.
Before we begin…
If you have not downloaded Android Studio, you can download it from here. We will also need JDK (Java Development Kit), which you can download from here, and we need Android SDK, which is already included with the Android Studio. After downloading and installing, we are more than ready to begin this lesson.
Creating a new project
After opening Android Studio for the first time, it will display a window where you can create or open a project, if it doesn’t appear let’s just create a new one by going to File > New > New Project, a screen like the following will appear:

Under “Application Name”, we will be writing how our application will be named and displayed as an app when we run it in our phones, I will be calling my application: “TextDisplayer”, and under “Company Domain”, we will write any name so that the package name follows this pattern: “com.companyname.appname”. Hit next after setting the project location path.
The next step will be choosing the targeted devices, phone, tablets, android wear, TV, auto, and Glass. You can select multiple devices, this will depend in your app’s focus. We will be selecting the Phone and Tablet only and now we need to select the minimum SDK for our app. We will be considering various factors: the lower the minimum SDK, the less features you will get, however, we will be targeting more devices. You will notice that there’s a percentage provided by the IDE, which allows you to check an estimate of the devices that runs in that specific version and later. I will be choosing API 19: Android 4.4 (KitKat), considering I will be running this in my phone (connected with USB Debugging On).

Now for the last step, we can choose an activity from the ones that are listed or add no activity. These activities are pre-configured and comes with Android Studio as templates to avoid “reinventing the wheel” and fasten the process of creating one of the listed activities. We will be looking at most of the activities later on, but now we will be choosing an empty activity, which means a page with absolutely nothing in it. You choose a name for that activity and click finish. This is how we can create a project in Android Studio.
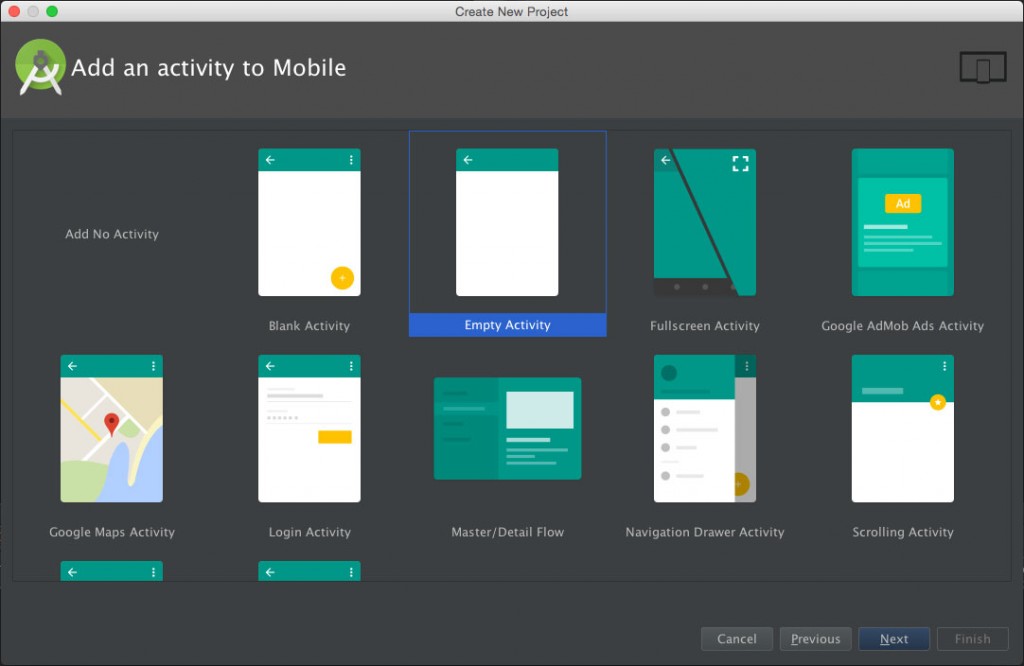
Let the gradle process finish and let’s start checking what files has been created. Under “manifests”, we can find AndroidManifest.xml, which basically functions as the configuration file for activities (all activities will be declared here), permissions (Internet, Contacts, etc.), and other related to the application. The java folder contains all the java classes and activitiesthat the application will use, right now we only have MainActivity, and we can find the JUnit Test Package. We will also find a resource folder that contains all the layouts, drawables, icons, and strings used by the application. If you ask yourself what’s under Gradle Scripts, you are very curious! Gradle Scripts contains properties, settings, dependencies, android SDK version, minimun SDK Version, and many other app-related settings. For example, you can add more dependencies like Google Gson, etc. in build.gradle (app).

What’s Next?
We have seen how to create a project, went through each folder and check what’s inside (not into detail), and we could also run the project by clicking play/run icon, requiring a smartphone with USB Debugging On or an emulated device.
In the next tutorial, we are going to detail the Activity class and define every method that you could use, XML files like the layouts, strings, etc.. Theory is always good!